Are you concerned about the security of your Node.js application? Are you aware of the potential risks and vulnerabilities it may face in today’s ever-evolving threat landscape? Protecting your application from malicious attacks and unauthorized access is crucial to safeguarding sensitive data and maintaining user trust. But where do you begin? Â
In a world where cyber threats continue to increase in frequency and sophistication, it’s essential to take proactive steps to secure your Node.js application. From code vulnerabilities to insecure configurations, there are numerous aspects to consider when it comes to application security. Â
In the past year alone, there has been a staggering 40% increase in reported security breaches targeting web applications. These attacks exploit vulnerabilities in applications, compromising sensitive data, damaging reputations, and resulting in significant financial losses for businesses. Â
In this blog, we will explore best practices and effective strategies for ensuring the security of your Node.js application. By addressing common security challenges and implementing robust security measures, you can significantly reduce the risk of breaches and protect your application from potential threats.Â
Secure Configuration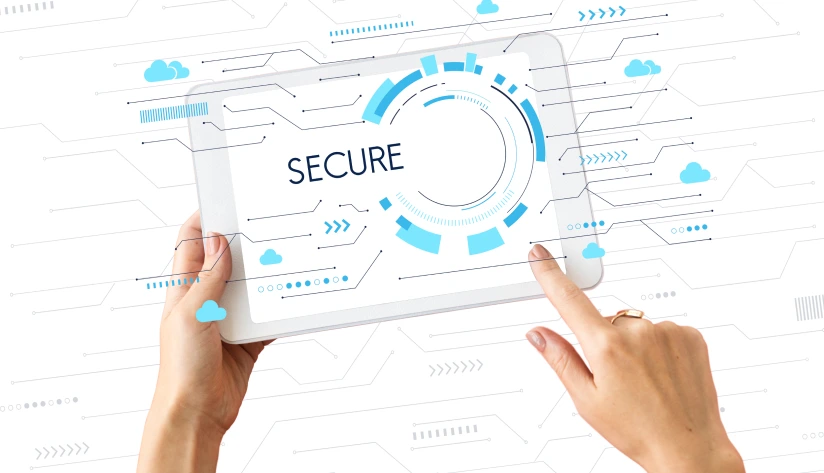
One of the foundational aspects of ensuring the security of a Node.js application is to establish a secure configuration. Proper configuration practices help mitigate potential vulnerabilities and reduce the risk of unauthorized access or data breaches. In this section, we will discuss key considerations for maintaining a secure configuration in your Node.js application.Â
- Keep Software Dependencies Updated:  Regularly updating the Node.js runtime and all third-party dependencies is crucial for maintaining a secure configuration. Outdated software may have known vulnerabilities that can be exploited by attackers. Keep track of updates and security patches for your dependencies and promptly apply them to mitigate potential risks.Â
- Securely Store Sensitive Data:  Storing sensitive data, such as API keys, database credentials, and encryption keys, requires careful consideration. Avoid hardcoding or storing these credentials directly in your application’s source code. Instead, use environment variables or a secure configuration management solution to store and retrieve sensitive information. This approach prevents accidental exposure and unauthorized access to critical data.Â
- Implement Access Controls:  Proper access controls are essential for protecting your application’s configuration. Limit access to sensitive files and configuration settings to authorized personnel only. Use role-based access control (RBAC) mechanisms to define and enforce granular permissions based on user roles and responsibilities. Strong password policies and the implementation of multi-factor authentication (MFA) can further enhance access control and security.Â
- Protect Configuration Files:  Configuration files often contain sensitive information that could be exploited if accessed by unauthorized individuals. Apply encryption to protect the confidentiality of these files. Restrict file permissions to ensure that only authorized users can access and modify them. Regularly monitor and review access rights to prevent unauthorized modifications and maintain the principle of least privilege.Â
- Employ Firewall and Network Security Measures:  Utilize firewalls to control incoming and outgoing network traffic for your Node.js application. Configure the firewall to allow access only to necessary ports and protocols, blocking unauthorized access attempts. Implement intrusion detection and prevention systems (IDS/IPS) to monitor network traffic and detect potential attacks in real time.Â
- Regularly Review and Test Configuration:  Perform regular reviews and audits of your application’s configuration settings. This helps identify misconfigurations and security gaps. Conduct configuration audits and penetration testing to assess the effectiveness of your security measures. Utilize static code analysis tools and vulnerability scanners to identify and address configuration-related vulnerabilities proactively.Â
Input Validation and Sanitization
Proper input validation and sanitization are crucial steps in securing a Node.js application. Failing to validate and sanitize user input can lead to vulnerabilities such as cross-site scripting (XSS) and SQL injection attacks. In this section, we will delve into the importance of input validation and sanitization, along with best practices to implement them effectively.Â
Why is Input Validation Important? Input validation ensures that the data provided by users meets the expected criteria. By validating user input, you can prevent malicious data from being processed by your application and potentially compromising its security. It helps maintain the integrity of your application’s data and prevents common vulnerabilities.Â
Best Practices for Input Validation and Sanitization
- Use Trusted Libraries or Frameworks:  Leverage trusted libraries or frameworks, such as Express Validator, to simplify the process of input validation. These tools provide built-in validation and sanitization methods, making it easier to handle user input securely.Â
- Implement Server-Side Validation:  Client-side validation is convenient for improving the user experience, but it should never be relied upon solely for security purposes. Always perform server-side validation to validate input data. Client-side validation can be bypassed, so server-side validation acts as the last line of defense.Â
- Define Strict Validation Rules:  Create strict validation rules based on the expected format and type of input. For example, if you expect an email address, validate it against a regular expression or use specific validation functions to ensure it conforms to the expected format.Â
- Sanitize User Input:  Sanitizing user input involves removing or encoding potentially dangerous characters to prevent XSS attacks and other forms of injection. Utilize libraries like DOMPurify or validator.js to sanitize user input effectively. These libraries help remove or escape malicious characters, ensuring that user input is safe for processing.Â
- Parameterized Queries and Prepared Statements:  When interacting with databases, utilize parameterized queries or prepared statements instead of building queries dynamically with user input. This approach prevents SQL injection attacks by separating the query logic from the user-supplied data.Â
- Validate File Uploads:  If your application allows file uploads, thoroughly validate and sanitize the uploaded files. Check file extensions, restrict file sizes, and use antivirus scanners to detect potential threats. Additionally, store uploaded files in a separate location outside the web root to prevent direct access to them.Â
Authentication and Authorization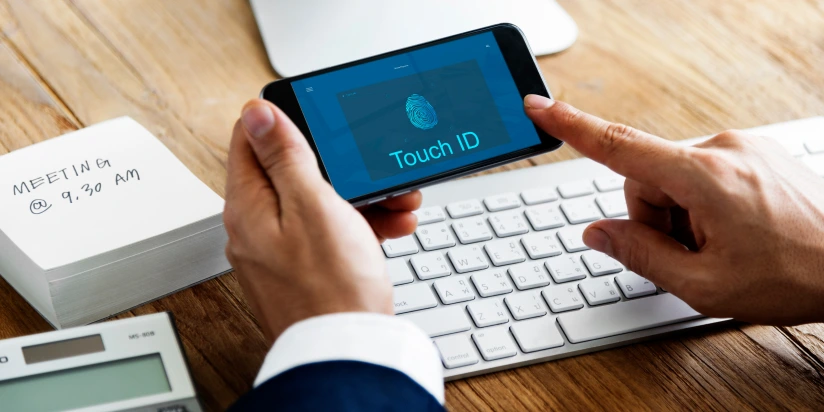
Authentication and authorization are essential components of a secure Node.js application. Authentication ensures that users are who they claim to be, while authorization determines what actions and resources they are allowed to access. In this section, we will explore best practices for implementing strong authentication and authorization mechanisms in your Node.js application.Â
- Secure Password Handling:  When it comes to authentication, storing user passwords securely is of utmost importance. Avoid storing plain-text passwords by utilizing strong hashing algorithms, such as bcrypt or Argon2, to securely hash and store passwords in your database. Implement techniques like salting and stretching to add an extra layer of protection against password-cracking attempts.Â
- Multi-Factor Authentication (MFA):  Implementing multi-factor authentication provides an additional layer of security by requiring users to verify their identity through multiple factors, such as a password, SMS code, or biometric authentication. Leverage libraries like Passport.js to integrate MFA seamlessly into your Node.js application.Â
- Use Secure Session Management:  Implement secure session management to maintain user sessions securely. Generate secure session tokens, such as JSON Web Tokens (JWT), and store them securely, either in memory or as HTTP-only cookies. Set appropriate session expiration times and implement mechanisms to detect and prevent session hijacking and session fixation attacks.Â
- Role-Based Access Control (RBAC):  Implement RBAC to manage and enforce user permissions and access rights. Define roles with specific sets of permissions and assign them to users based on their responsibilities. Use middleware or custom authorization logic to verify user roles and restrict access to certain resources or actions based on their assigned roles.Â
- Protect Against Cross-Site Request Forgery (CSRF):  Prevent CSRF attacks by implementing CSRF protection mechanisms. Generate and validate CSRF tokens for every user request that modifies data or performs sensitive actions. Include CSRF tokens as hidden fields in HTML forms or as custom headers in AJAX requests to validate the authenticity of the requests.Â
- Securely Manage User Sessions:  Protect user sessions from session-related vulnerabilities. Ensure that session tokens are securely transmitted over encrypted connections (HTTPS) to prevent eavesdropping or interception. Implement session regeneration after significant events like authentication, authorization changes, or privilege escalation to prevent session fixation attacks.Â
- Logging and Monitoring:  Implement logging and monitoring mechanisms to track and detect any suspicious activities related to authentication and authorization. Log authentication and authorization events failed login attempts and unauthorized access attempts. Utilize logging frameworks like Winston and implement real-time monitoring solutions to identify potential security breaches promptly.Â
Handling and Logging ErrorsÂ
Proper error handling and logging are essential aspects of maintaining the security and stability of a Node.js application. By effectively handling errors, you can prevent sensitive information from being exposed and maintain the integrity of your application. In this section, we will discuss best practices for error handling and logging in your Node.js application.Â
- Graceful Error Handling:  Learn how to handle unexpected errors and exceptions gracefully to prevent information leakage and maintain a positive user experience.Â
- Centralized Error Handling:  Implement a centralized approach to handle errors consistently throughout your application, ensuring standardized error responses.Â
- Secure Logging:  Discover how to securely log error information, including relevant contextual data, while safeguarding sensitive information.Â
- Differentiating Operational and Programmer Errors:  Understand the distinction between operational errors and programmer errors, and handle them appropriately to minimize risk.Â
- Monitoring and Alerting:  Set up monitoring and alerting mechanisms to proactively detect critical errors and promptly respond to potential security-related issues.Â
- Regular Review of Error Logs:  Learn the importance of regularly reviewing and analyzing error logs to identify patterns, security vulnerabilities, and areas for improvement.Â
Cross-Site Scripting (XSS) and Cross-Site Request Forgery (CSRF) Prevention
Cross-Site Scripting (XSS) and Cross-Site Request Forgery (CSRF) are common web application vulnerabilities that can compromise the security of your Node.js application. In this section, we will explore best practices for preventing XSS and CSRF attacks, helping you safeguard your application against these threats.Â
- Cross-Site Scripting (XSS) Prevention:  Learn how to protect your Node.js application from XSS attacks by implementing proper input sanitization, output encoding, and content security policies.Â
- Input Sanitization:  Understand the importance of validating and sanitizing user input to prevent malicious scripts from being executed on your application.Â
- Output Encoding:  Implement proper output encoding techniques to ensure that user-generated content is displayed safely, mitigating the risk of XSS vulnerabilities.Â
- Content Security Policies (CSP):  Utilize Content Security Policies to define a set of rules that specify which content is allowed to be loaded by your application, reducing the likelihood of XSS attacks.Â
- Cross-Site Request Forgery (CSRF) Prevention:  Discover methods to protect your Node.js application against CSRF attacks, including the use of CSRF tokens and proper request validation.Â
- CSRF Tokens:  Implement CSRF tokens to verify the authenticity of requests and prevent attackers from forging requests on behalf of authenticated users.Â
- SameSite Cookies:  Utilize the SameSite attribute for cookies to restrict their usage to same-site requests, preventing CSRF attacks that rely on cookie-based authentication.Â
- Request Validation:  Implement request validation techniques to ensure that requests originate from trusted sources, protecting your application from unauthorized CSRF attempts.Â
Secure Communication Â
Securing communication is crucial for protecting sensitive data transmitted over networks in your Node.js application. In this section, we will explore best practices for ensuring secure communication, including encryption, authentication, and secure protocols, to safeguard data integrity and confidentiality.Â
- Transport Layer Security (TLS):  Learn the importance of implementing TLS encryption to secure communication between your Node.js application and clients. Configure TLS certificates and enforce HTTPS to protect data from interception and tampering.Â
- Certificate Management:  Understand how to properly manage TLS certificates, including certificate issuance, renewal, and revocation. Regularly update certificates to maintain strong encryption and trust.Â
- Strong Cipher Suites and Protocols:  Configure your Node.js application to use strong cipher suites and protocols, such as TLS 1.3, to ensure secure communication. Disable outdated and vulnerable protocols like SSLv3 and TLS 1.0/1.1 to mitigate potential risks.Â
- Authentication and Authorization:  Implement strong authentication mechanisms, such as client certificates, OAuth, or JSON Web Tokens (JWT), to verify the identities of clients and authorize access to sensitive resources. Use secure protocols like OAuth 2.0 for delegated authorization.Â
- Secure WebSocket Communication:  If your application utilizes WebSocket for real-time communication, ensure secure WebSocket connections by employing TLS encryption. Secure WebSocket communication prevents eavesdropping and ensures data privacy.Â
- API Security:  When developing APIs in your Node.js application, consider implementing secure practices such as rate limiting, input validation, and authorization checks. Protect API endpoints from unauthorized access and potential attacks.Â
- Security Headers:  Utilize security headers, such as Content Security Policy (CSP), Strict-Transport-Security (HSTS), and X-XSS-Protection, to enhance the security of your application. These headers provide additional layers of protection against common web vulnerabilities.Â
Regular Security Audits and Penetration Testing
Regular security audits and penetration testing are essential for identifying vulnerabilities and weaknesses in your Node.js application’s security posture. By conducting thorough assessments, you can proactively address security issues and enhance the overall resilience of your application. In this section, we will explore the importance of security audits and penetration testing, along with best practices for conducting them effectively.Â
- Security Audits:  Understand the significance of regular security audits to evaluate your Node.js application’s security controls, configurations, and adherence to industry best practices. Audits help identify potential vulnerabilities, compliance gaps, and areas for improvement.Â
- Conducting Penetration Testing:  Learn about the importance of penetration testing in assessing the effectiveness of your application’s security defenses. Penetration tests simulate real-world attacks to uncover vulnerabilities that could be exploited by malicious actors.Â
- Engage Security Professionals:  Consider involving experienced security professionals or external security firms to conduct security audits and penetration tests. Their expertise and knowledge can provide valuable insights and help identify security risks that may be overlooked internally.Â
- Identify Potential Attack Vectors:  Collaborate with security experts to identify potential attack vectors specific to your Node.js application. This includes reviewing code, configurations, network infrastructure, and user access controls to assess areas vulnerable to exploitation. Â
- Vulnerability Scanning:  Utilize automated vulnerability scanning tools to identify common vulnerabilities in your application. These tools can help detect security weaknesses related to outdated libraries, known vulnerabilities, or misconfigurations.Â
- Security Patch Management:  Establish a robust security patch management process to ensure the timely installation of security patches and updates for your application’s dependencies. Regularly update and patch software components to address known vulnerabilities.Â
- Secure Deployment Practices:  Implement secure deployment practices, such as using secure configurations, securely managing environment variables and secrets, and minimizing the attack surface by only enabling necessary services and ports.Â
- Remediation and Follow-Up:  Actively address identified vulnerabilities and weaknesses through proper remediation efforts. Develop a plan to prioritize and resolve issues discovered during security audits and penetration testing. Follow up with post-remediation validation to ensure effectiveness.Â
Conclusion Â
Securing your Node.js application is a continuous process that requires a proactive approach. By following the best practices outlined in this blog, you can significantly enhance the security of your application and protect it from potential threats. Remember to stay updated on emerging security trends and regularly review and update your security measures. By prioritizing security, you can ensure the trust and confidence of your users while safeguarding your application from malicious activities.
Want more information about our services?